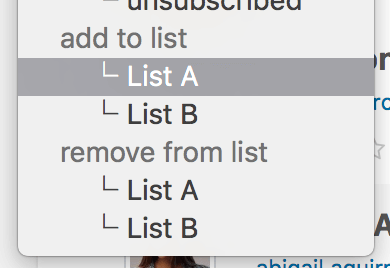
It’s easy to assign subscribers to the list with the UI of Mailster. Just select the subscribers you like to assign and select the option from the drop-down. To get more specific you need to add some custom code to your website.
There are many situations where you like to move a user to a certain list. In any case, you need two methods:
mailster( 'subscribers' )->assign_lists( $subscriber_ids, $lists, $remove_others, $confirm );
to add lists to your subscribers and
mailster( 'subscribers' )->unassign_lists( $subscriber_ids, $lists );
$subscriber_ids
can either be a single ID of one of your subscribers or an array of IDs.
$lists
can either be a single ID of one of your lists or an array of IDs.
Assign a list after clicking a link
Let’s assign the subscriber who clicks on the link to “http://example.com” to the List with ID 1.
add_action( 'mailster_click', function( $subscriber_id, $campaign_id, $target, $index ) {
$list_id = 1;
$remove_others = false;
$confirmed = true;
if ( $target === 'http://example.com' ) {
mailster( 'subscribers' )->assign_lists( $subscriber_id, $list_id, $remove_others, $confirmed );
}
}, 10, 4);
Assign two lists after opening a specific campaign
Let’s assign the subscriber from a specific campaign (ID 12) to the List with ID 1 and 2 one the campaign was opened.
add_action( 'mailster_open', function( $subscriber_id, $campaign_id ) {
$list_id = array(1, 2);
$remove_others = false;
$confirmed = true;
if ( $campaign_id == 12 ) {
mailster( 'subscribers' )->assign_lists( $subscriber_id, $list_id, $remove_others, $confirmed );
}
}, 10, 2);
Assign two lists after a specific campaign was sent
Let’s assign the subscriber to the List with IDs 1 and 2 when a specific campaign (ID 12) has been sent.
add_action( 'mailster_send', function( $subscriber_id, $campaign_id, $result ) {
$list_id = array(1, 2);
$remove_others = false;
$confirmed = true;
if ( $campaign_id == 12 ) {
mailster( 'subscribers' )->assign_lists( $subscriber_id, $list_id, $remove_others, $confirmed );
}
}, 10, 3);
Moving a subscriber from one list to another after a certain action
Since we don’t know the current subscriber we have to get it from the current WordPress User. This requires an existing WordPress user with a matching Mailster Subscriber.
add_action( 'my_custom_action', function( ) {
if( $subscriber = mailster( 'subscribers' )->get_by_wpid() ){
$list_id_from = 1;
$list_id_to = 2;
$remove_others = false;
$confirmed = true;
mailster( 'subscribers' )->unassign_lists( $subscriber->ID, $list_id_from );
mailster( 'subscribers' )->assign_lists( $subscriber->ID, $list_id_to, $remove_others, $confirmed );
}
});
Add subscribers to a list after a certain campaign has been sent.
Maybe you like to move a subscriber to a different list if he got a certain campaign.
In this example, any subscriber where the campaign with the id “123” has been sent will be added to the list with id “3”.
add_action( 'mailster_send', function( $subscriber_id, $campaign_id ) {
if( $campaign_id == 123 ){
$list_id_to = 3;
$remove_others = false;
$confirmed = true;
mailster( 'subscribers' )->assign_lists( $subscriber_id, $list_id_to , $remove_others, $confirmed);
}
}, 10, 2);
You can replace “mailster_send
” with
mailster_open
to move when the campaign has been opened.mailster_click
to move when the campaign has been clicked.