Sometimes you’re in the need to add some custom dynamic content to your newsletters. You may use it for coupon codes, custom status, or even the weather in a specific region. To achieve that you can start with this snippet
add_action( 'mailster_add_tag', function() {
mailster_add_tag('mytag', function( $option, $fallback, $campaignID = null, $subscriberID = null ) {
return 'My Tag: Option: ' . $option . '; Fallback: ' . $fallback;
});
});
If you add this to your themes function.php you can now use {mytag}
in your newsletters.
If you like to add options to the tag like {mytag:my_option}
you find this value in the $option
variable
To define a fallback value in your tag use {mytag|my_fallback}
or {mytag:my_option|my_fallback}
to add ‘my_fallback’ in the $fallback
variable.
Once the tag is added you can select it from the drop-down menu in the editor:
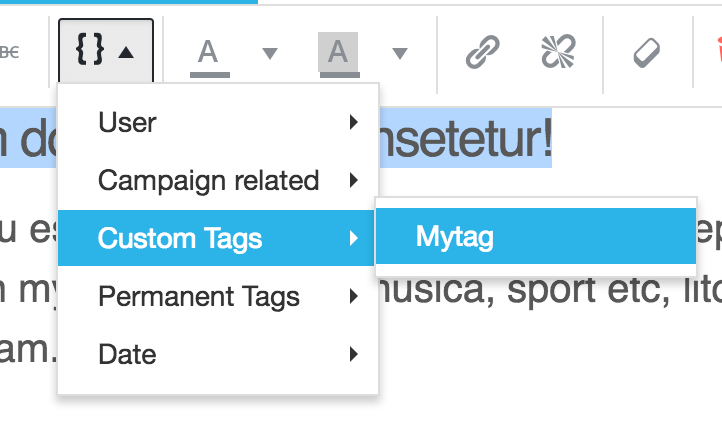
Using different content for each subscriber
Sometimes you like to display unique content for each subscriber which you don’t like to store in custom fields or create on demand. Here’s an example of how to use coupon codes in a dynamic tag.
Create a source
First, you have to define the source which your data is coming from. In our case, it’s a function that generates the code but it can be a database table or a simple array.
Let’s create a get_subscriber_coupon
the method which needs the subscriber ID to get the same unique code for each individual subscriber.
function get_subscribers_coupon( $subscriber_id ) {
$seed = AUTH_SALT;
$length = 10;
$code = substr( strtoupper( base_convert( md5( $seed . $subscriber_id ), 16, 36 ) ), 0, $length );
return $code;
}
This method creates a 10-character long, uppercase string based on the subscriber ID. It uses the AUTH_SALT
constant as a seed so you don’t get the same code on every website.
You should create your own custom method if you like to make sure nobody can just guess your coupon code.
The dynamic tag
Now we need a dynamic tag that we can use in our campaign to display the generated code. We create a new function for a {coupon}
tag.
add_action( 'mailster_add_tag', function() {
mailster_add_tag( 'coupon', function( $option, $fallback, $campaignID = null, $subscriberID = null ) {
// make sure the subscriber ID is set
if ( ! is_null( $subscriberID ) ) {
return get_subscribers_coupon( $subscriberID );
}
// return the fallback "NOCOUPONCODE4U"
return $fallback;
} );
});
This method will first check if a subscriber is set and will return the code for this subscriber. If not set it will return the $fallback
which is an empty string by default or the content you define in your code.
You can now use the following code in your campaigns:
{coupon}
=> no fallback will be displayed
{coupon|NOCOUPONCODE4U}
=> “NOCOUPONCODE4U” will be displayed if no user is defined
You can also hardcode a fallback into your function if you prefer this.
This is a very basic example of getting custom content for your subscribers.